This post explain how permissions work with Amazon S3 in regard to Asp.net MVC application.
We will create a bucket and a folder (UPLOADS), and then we’ll create AWS Identity and AMI user in your AWS account and grant permissions on your Amazon S3 bucket and the folders
The sample code is available for anyone to use
I.Creating .net MVC app
You can use visual sudio to create your MVC app. For more info on how to create an application on Microsoft site. For this demo we are using Visual studio 2017
- Open Visual Studio.
- Click File > New > Project or press CTRL+SHIFT+N.
- In the installed templates select ASP.net web Application (.Net Framework) under Web in your left pane
- Give it a name and click Ok
- Select MVC and change authentication to Individual user account then click OK.
When you press F5 you should be able to register and login in your app.
II. Adding S3 references to your project
To access S3 functionalities you will need tinstall the following:
AWS SDK for .NET
NuGet is a package management system for the .NET platform. With NuGet, you can add the AWSSDK assemblies . So add this by right clicking your project, Manage Nuget packahges , then Browse. look for AWSSDK for .NET.
This assembly will allow you to add the
using Amazon.S3; and using Amazon;
In the manage controller let set some properties for amazon Key Access:
private static IAmazonS3 s3Client;
private string _awsAccessKey = ConfigurationManager.AppSettings["AWSAccessKey"];
private string _awsSecretKey = ConfigurationManager.AppSettings["AWSSecretKey"];
private string _bucketName = ConfigurationManager.AppSettings["AWSProfileName"];
<add key="AWSProfileName" value="bucket name" />
<add key="AWSAccessKey" value="your Aws access key" />
<add key="AWSSecretKey" value="Aws secret key />
Now let go back to AWS console to create the keys
We assume you already have AWS account. You can have a free tier account from Amazon and use it for one year FREE
- Step 1: Create a Bucket
- Go to AWS S3 after login,
- create Bucket name dotnetbucket.uploads
- make sure that you block public access(Meaning use the default setting from aws)
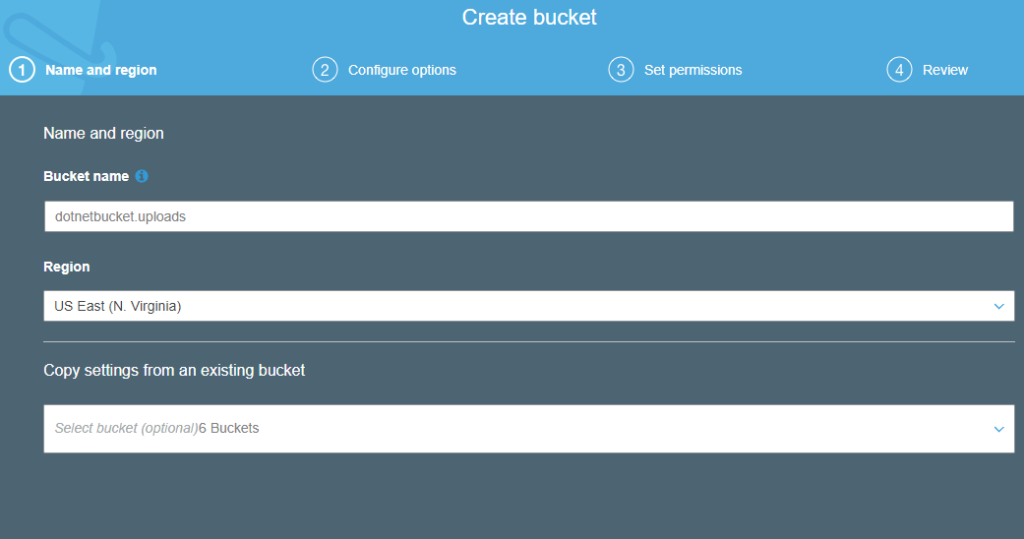
- Final screen
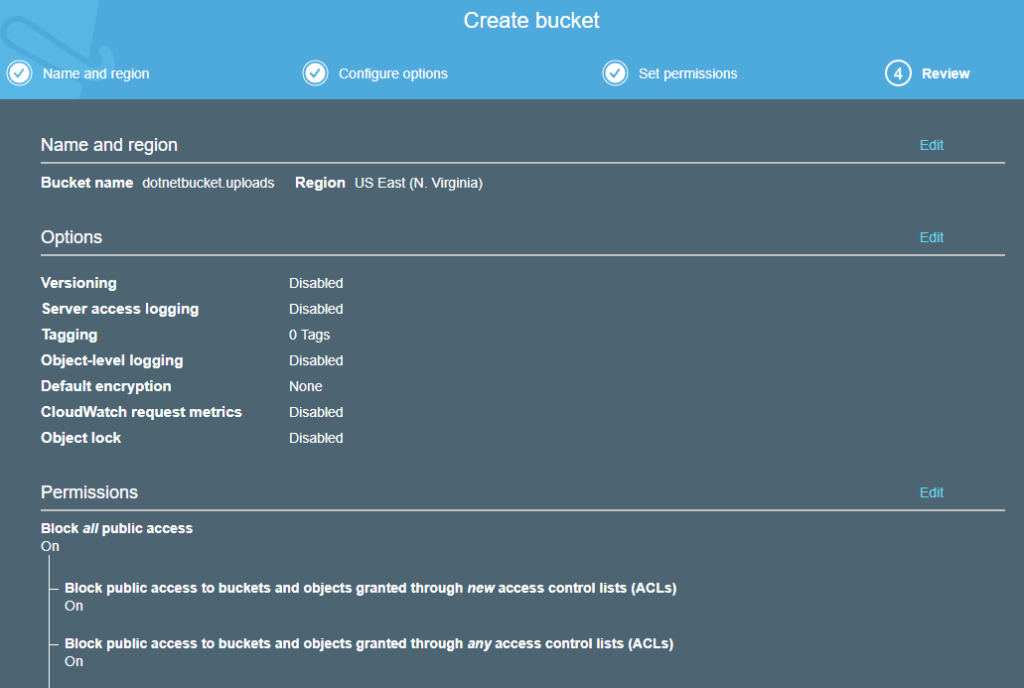
- Step 2: Create IAM Users and a Group
- Go to IAM console to add an IAM user name dotnetbucketwebuser and group called dotnetbucket . make sure to check Access Type to Programmatic access
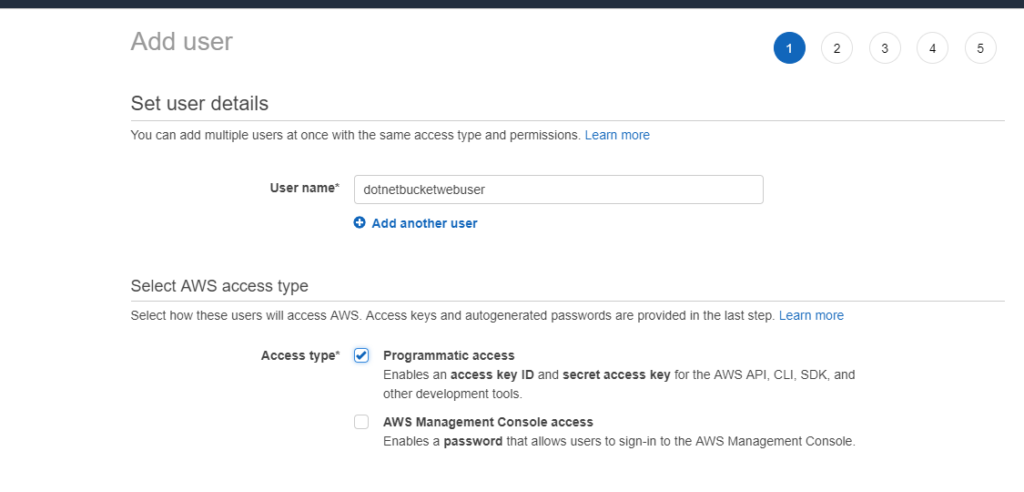
- In the next step, create the group dotnetbucket to add the user
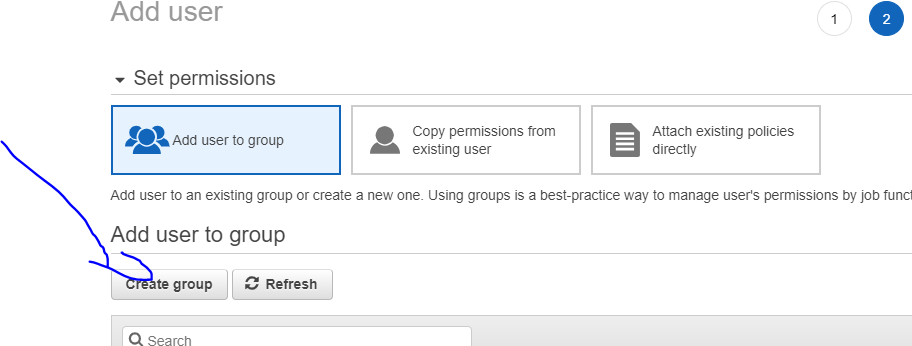
- Then create the group with the name and then create user. At this point you can see that the user does not have access to the bucket created
- Step 3 You can see that the new created user has no permission to see UPLOADS bucket . You can simply verify that by login in another browser with the newly created user and browse https://console.aws.amazon.com/s3/ . you will see the error below
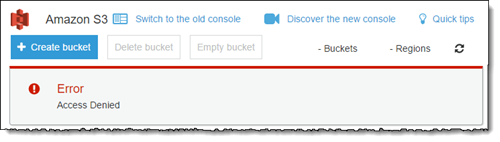
Now we have many ways to give the permission to the group or user we have created. For this demo, we will show how to generate IAM policies and assign to the group for the created Bucket using the Visual feature. You can also generate it with the Json tab.
Here is the steps to create IAM policy:
- Go to S3
- Select the bucket we have created
- Go to permission tab then bucket policy tab
- Click on Policy generator
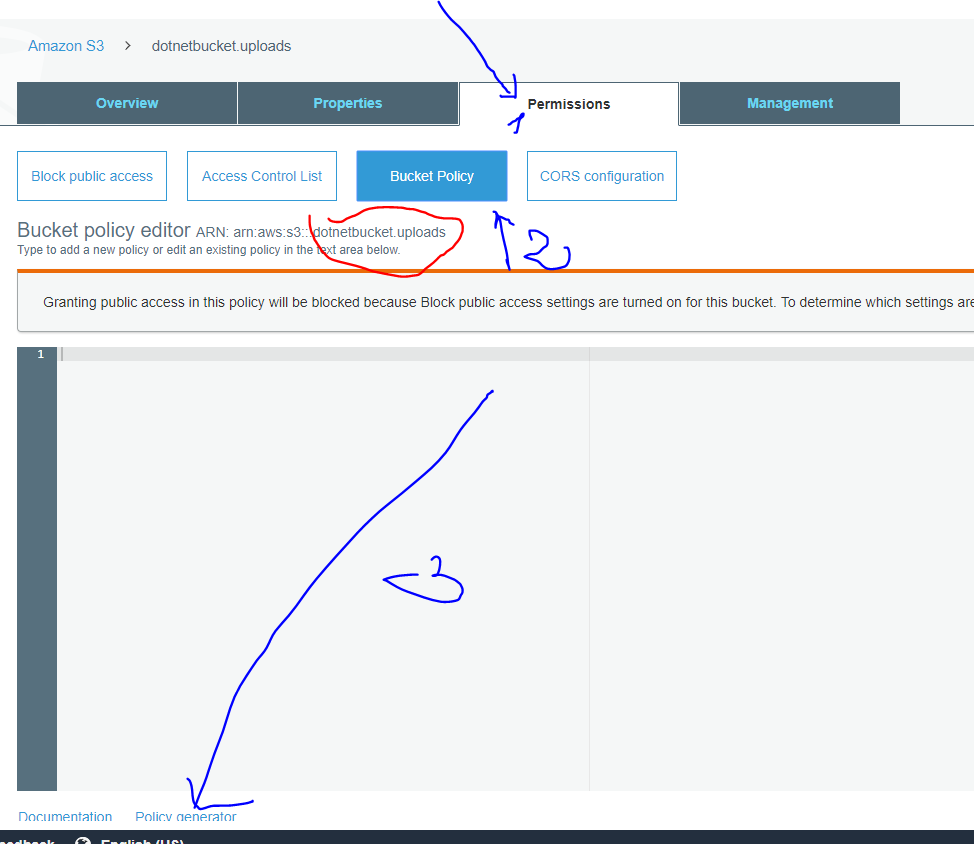
- Generate the olicy for PutObject, GetObject and delete Under Action
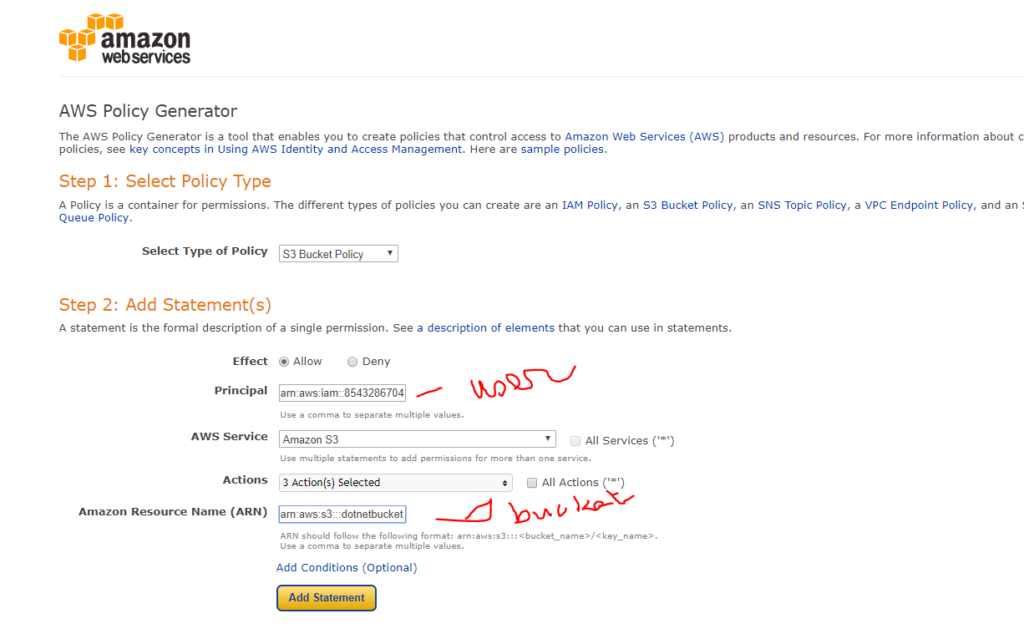
Click Add statement and you will see the below sumary. Then click on generate policy
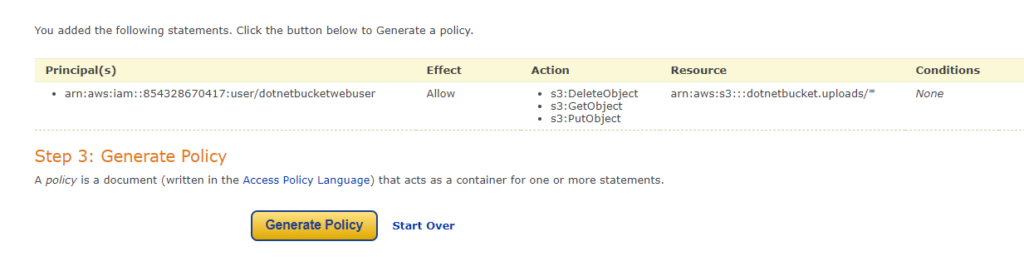
Copy that json and go back to the permission screen and paste it.Then press save
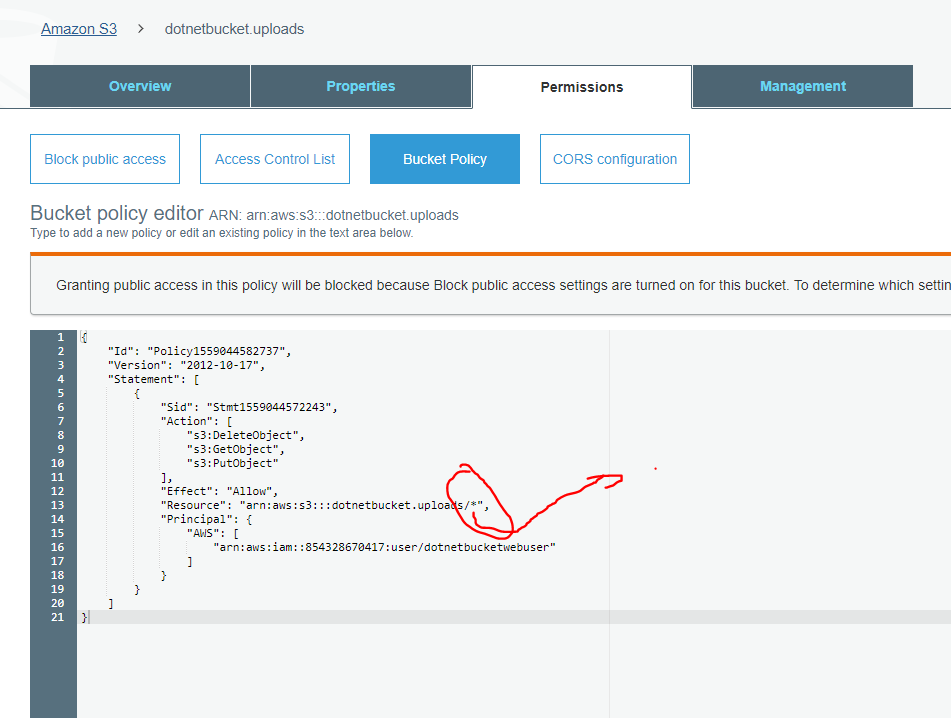
Attaching the policy to a group
- In the list of policies, select the check box next to the name of the policy to attach. You can use the Filter menu and the search box to filter the list of policies.
- Choose Policy actions, and then choose Attach.
- Select one or more identities to attach the policy to. You can use the Filter menu and the search box to filter the list of principal entities. After selecting the identities, choose Attach policy.
- We will chose the Group DotNetBucket and attach the policy
- That is it
At this point you should be able to upload image to the profile. You can add more function for crop image using for example Lamda functions from aws
Source code available in Git